Last week, we took a look at Aspire in general and how a empty Aspire solution looks like. This week, we'll take the next step by building three key components for our solution: an API, a Blazor frontend, and a shared library for managing data models with Entity Framework. Additionally, we'll integrate PostgreSQL as our database for robust data handling.
You can find the complete code for this project on GitHub: Aspire ToDo Repository.
Adding new Projects
Let's kick things off by creating the core projects for our solution. Run the following commands in your terminal to set up the necessary components:
dotnet new classlib -o ToDoApp.Data
dotnet new web -o ToDoApp.ApiService
dotnet new blazor -o ToDoApp.Web
dotnet sln .\ToDoApp.sln add .\ToDoApp.Data\ToDoApp.Data.csproj
dotnet sln .\ToDoApp.sln add .\ToDoApp.ApiService\ToDoApp.ApiService.csproj
dotnet sln .\ToDoApp.sln add .\ToDoApp.Web\ToDoApp.Web.csproj
These commands generate three projects and link them to the solution.
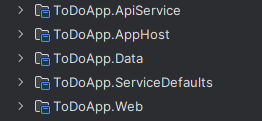
Now that we have created the projects and added them to our solution, let's dive into the purpose of each project and set them up to work together.
Understanding the project structure
ToDoApp.Data
The ToDoApp.Data
project serves as the foundation of our application. It houses the data models and Entity Framework configurations that will be shared between the API and frontend components, ensuring consistency and modularity.
ToDoApp.ApiService
The API project will expose endpoints for our ToDo application. This is where we'll add controllers, services, and any logic for interacting with the database.
ToDoApp.Web
The Blazor frontend project will be the user interface for our application. It will communicate with the API to display and manage ToDo items interactively.
Adjustments to the AppHost project
Registration of PostgresSQL
To add a PostgreSQL Database to our project, we can simply register it in the AppHost
project.
dotnet add .\ToDoApp.AppHost\ToDoApp.AppHost.csproj packag Aspire.Hosting.PostgreSQL
This package provides extension methods and resource definitions to configure a PostgreSQL resource. Aspire's PostgreSQL extensions automate many tasks like Docker container creation, making it easier to set up a database without manual intervention.
For development purpose, it will automatically create a PostgreSQL Database Server with random credentials. Those random credentials will be mounted to your linked projects too.
To add the Database and create the Docker container on your local machine, it's sufficient to add the following code Program.cs
of the AppHost
project:
var postgres = builder.AddPostgres("postgres");
var postgresDb = postgres.AddDatabase("postgresdb");
For enhanced development convenience, you can also integrate pgAdmin to manage your PostgreSQL database visually:
if (builder.Environment.IsDevelopment())
{
postgres = postgres.WithPgAdmin();
}
API Service
Let's add the API Service to our Orchestration too. We pass a reference to the postgres database and wait for it to be ready.
var apiService = builder.AddProject<Projects.ToDoApp_ApiService>("api")
.WithReference(postgresDb)
.WaitFor(postgres);
Web Service
Lastly the only project we need to hook up to our Orchestration is the web project. You can do this by adding the following code to the AppHost
:
builder.AddProject<Projects.ToDoApp_Web>("web")
.WithExternalHttpEndpoints()
.WithReference(apiService)
.WaitFor(apiService);
Setting Up the Shared Class Library
To be able to use the Entity Framework, we have to add the NuGet Package Microsoft.EntityFrameworkCore
to the project.
dotnet add .\ToDoApp.Data\ToDoApp.Data.csproj package Microsoft.EntityFrameworkCore
First, let's define a basic entity in ToDoApp.Data
. Open the ToDoApp.Data
project and create two folders named Contexts
and Models
. Add a new file to Contexts
named ToDoContext.cs
and a file named ToDoItem.cs
to Models
. Add the following code to the ToDoItem.cs
:
[PrimaryKey(nameof(Id))]
public sealed class ToDoEntry
{
public int Id { get; set; }
[MaxLength(200)]
[Required]
public string? Title { get; set; } = string.Empty;
public bool? IsComplete { get; set; }
}
We've used data annotations to enforce validations and constraints. We specifically tell Entity Framework the name of the primary key of this dataset. To keep the data models simple and flexible, we're using nullable booleans and strings. This design choice allows us to reuse the model while conditionally modifying ToDo item properties based on the specifics of each request.
Now, let's configure the ToDoContext
class in the Contexts
folder. This class will act as the bridge between our application and the database. Add the following code to ToDoContext.cs
:
public class ToDoContext(DbContextOptions options) : DbContext(options)
{
public DbSet<ToDoEntry> ToDos { get; set; }
}
Configuring the API Project
With the shared library ready, it's time to integrate it into the API. In the ToDoApp.ApiService project, add a reference to the ToDoApp.Data project:
dotnet add .\ToDoApp.ApiService\ToDoApp.ApiService.csproj reference .\ToDoApp.Data\ToDoApp.Data.csproj
The Program.cs
file in the ToDoApp.ApiService
project orchestrates the API's behavior and establishes a seamless connection to the PostgreSQL database. Below is the complete configuration:
var builder = WebApplication.CreateBuilder(args);
builder.AddServiceDefaults();
builder.Services.AddProblemDetails();
builder.Services.AddOpenApi();
builder.AddNpgsqlDbContext<ToDoContext>("postgresdb");
var app = builder.Build();
app.UseExceptionHandler();
if (app.Environment.IsDevelopment())
{
app.MapOpenApi();
using var scope = app.Services.CreateScope();
var todoContext = scope.ServiceProvider.GetRequiredService<ToDoContext>();
todoContext.Database.EnsureCreated();
}
app.MapGet("/todos", ([FromServices] ToDoContext db) =>
{
var todos = db.ToDos.ToList();
return Results.Json(todos);
});
app.MapGet("/todos/{id}", ([FromServices] ToDoContext db, int id) =>
{
var todo = db.ToDos.Find(id);
if (todo == null)
{
return Results.NotFound();
}
return Results.Json(todo);
});
app.MapPost("/todos", ([FromServices] ToDoContext db, ToDoEntry todo) =>
{
todo.IsComplete ??= false;
if (todo.Title == null)
{
return Results.BadRequest();
}
db.ToDos.Add(todo);
db.SaveChangesAsync();
return Results.Created();
});
app.MapPatch("/todos/{id}", ([FromServices] ToDoContext db, [FromRoute] int id, [FromBody] ToDoEntry entry) =>
{
var todo = db.ToDos.Find(id);
if (todo == null)
{
return Results.StatusCode(304);
}
if (entry.IsComplete != null)
{
todo.IsComplete = entry.IsComplete;
}
if (!string.IsNullOrEmpty(entry.Title))
{
todo.Title = entry.Title;
}
db.SaveChanges();
return Results.NoContent();
});
app.MapDelete("/todos/{id}", ([FromServices] ToDoContext db, int id) =>
{
var todo = db.ToDos.Find(id);
if (todo == null)
{
return Results.NotFound();
}
db.ToDos.Remove(todo);
db.SaveChanges();
return Results.NoContent();
});
app.MapDefaultEndpoints();
app.Run();
What This Code Does
-
Configuring the Builder
builder.AddServiceDefaults()
: Adds default services commonly used in APIs.builder.Services.AddProblemDetails()
: Adds support for standardized error responses.builder.Services.AddOpenApi()
: Configures OpenAPI/Swagger for API documentation.builder.AddNpgsqlDbContext<ToDoContext>("postgresdb")
: Configures the database context to use PostgreSQL, where "postgresdb" is the connection string.
-
Ensuring Database Initialization
- In development mode, the app ensures the database schema is created using
EnsureCreated()
.
- In development mode, the app ensures the database schema is created using
-
Defining API Endpoints
- GET
/todos
: Fetches all ToDo entries as a JSON array. - GET
/todos/{id}
: Fetches a specific ToDo entry by its ID. Returns 404 if not found. - POST
/todos
: Adds a new ToDo entry. Automatically sets IsComplete to false if not provided. - PATCH
/todos/{id}
: Updates an existing ToDo entry partially. Returns 304 if the entry is not found. - DELETE
/todos/{id}
: Deletes a ToDo entry by its ID. Returns 404 if the entry doesn't exist.
- GET
Building the Blazor Frontend
To create an interactive user interface for our ToDo App, we've set up a Blazor project. This section will walk through the creation of a client to interact with the API and a Razor view to display and manage ToDo items.
Setting Up the Blazor Project
The ToDoApiClient
acts as a service layer for communicating with the backend API. Here's the implementation:
public class ToDoApiClient(HttpClient client)
{
public async Task<IEnumerable<ToDoEntry>> GetToDos(int maxItems = 10, CancellationToken cancellationToken = default)
{
List<ToDoEntry> toDoEntries = [];
await foreach (var toDo in client.GetFromJsonAsAsyncEnumerable<ToDoEntry>("/todos", cancellationToken))
{
if (toDoEntries.Count >= maxItems)
{
break;
}
if (toDo is not null)
{
toDoEntries.Add(toDo);
}
}
return toDoEntries;
}
public async Task<ToDoEntry?> GetToDo(int id)
{
var toDo = await client.GetFromJsonAsync<ToDoEntry>($"/todos/{id}");
return toDo;
}
public async Task<HttpResponseMessage> AddToDo(ToDoEntry toDo)
{
return await client.PostAsJsonAsync("/todos", toDo);
}
public async Task<HttpResponseMessage> PatchToDo(ToDoEntry toDo)
{
return await client.PatchAsJsonAsync($"/todos/{toDo.Id}", toDo);
}
public async Task<HttpResponseMessage> DeleteToDo(int id)
{
return await client.DeleteAsync($"/todos/{id}");
}
}
To use the client, we register it in our Program.cs
:
builder.Services.AddHttpClient<ToDoApiClient>(client =>
{
client.BaseAddress = new Uri("https+http://api");
});
Implementing the ToDo Razor View
The ToDos.razor
component is responsible for displaying and managing the ToDo list. Below is the complete implementation of the ToDo view. While simple in design, it effectively demonstrates core functionality.:
@page "/todos"
@rendermode InteractiveServer
@using ToDoApp.Data.Models
@inject ToDoApiClient ToDoApiClient
<PageTitle>ToDo List</PageTitle>
<h1>ToDo List</h1>
@if (_toDos == null)
{
<p>
<em>
Loading...
</em>
</p>
}
else
{
<input type="text" placeholder="Add a new ToDo" @bind="_newToDoTitle" />
<button @onclick="async () => await AddTodo()">Add</button>
<ul>
@foreach (var toDo in _toDos)
{
<li>
<input value="@toDo.Title" @onchange="async (args) => await UpdateTodoTitle(args, toDo)"/>
<input type="checkbox" checked="@(toDo.IsComplete != null && toDo.IsComplete.Value)" @oninput="async (args) => await UpdateTodoChecked(args, toDo)" />
<span style="cursor: pointer" @onclick="async () => await DeleteTodo(toDo)">❌</span>
</li>
}
</ul>
}
@code {
private IEnumerable<ToDoEntry>? _toDos;
private string? _newToDoTitle;
private async Task UpdateToDos()
{
_toDos = await ToDoApiClient.GetToDos();
}
protected override async Task OnInitializedAsync()
{
await UpdateToDos();
}
private async Task AddTodo()
{
if (!string.IsNullOrEmpty(_newToDoTitle))
{
await ToDoApiClient.AddToDo(new ToDoEntry { Title = _newToDoTitle, IsComplete = false });
await UpdateToDos();
}
}
private async Task UpdateTodoChecked(ChangeEventArgs args, ToDoEntry toDo)
{
toDo.IsComplete = args.Value as bool?;
if (!string.IsNullOrEmpty(toDo.Title))
{
await ToDoApiClient.PatchToDo(toDo);
}
}
private async Task UpdateTodoTitle(ChangeEventArgs args, ToDoEntry toDo)
{
toDo.Title = args.Value as string;
if (!string.IsNullOrEmpty(toDo.Title))
{
await ToDoApiClient.PatchToDo(toDo);
}
}
private async Task DeleteTodo(ToDoEntry toDo)
{
await ToDoApiClient.DeleteToDo(toDo.Id);
await UpdateToDos();
}
}
Wrapping Up
With the Blazor frontend in place, our ToDo application is now fully functional. The frontend interacts seamlessly with the API, offering a clean and responsive user experience.
In this post, we covered:
- Setting up the ToDoApiClient for API communication.
- Creating a dynamic Razor component for managing ToDo items.
- Integrating the Blazor project with the backend.
With the core functionality in place, this app serves as a solid foundation for enhancements like authentication, real-time updates, and deployment. In upcoming posts, we'll explore these advanced features to take the application to the next level. Stay tuned!